Variables
“ Variable is a name of memory location “
int data=123;
Variable is a symbolic name refer to a memory location used to store values that can change during the execution of a program.
Example:
int a = 10;
Here, int = data type
a = identifier
10 = literal
● There are three types of variables in java: local, instance and static.
1) Local Variable
A variable which is declared inside the method is called local variable.
2) Instance Variable
A variable which is declared inside the class but outside the method, is called instance variable . It is not declared as static.
3) Static variable
A variable that is declared as static is called static variable. It cannot be local.
Demo:-
class A
{
int data=50;//instance variable
static int m=100;//static variable
void method()
{
int n=90;//local variable
}
}
Data Types:-
● There are two types of data types in java: primitive and non-primitive.
● Primitive data types are the basic blocks of any programming language.
● It can have only one value at a time.
Data Types:-
● Non primitive data types are also referred to as derived types.
● Java supports non primitive data types classes, interfaces, and arrays.
Identifiers:-
● Are names assigned to variables, methods,constant,classes,packages and interfaces.
● No limit has been specified for length.
● Rules:
1. first letter must be a letter,underscore,dollar sign
2. subsequent can be letter,underscore,dollar,digit
3. white space is not allowed
4. identifiers are case sensitive
5. don't use keywords.
Naming Convention:-
● Class or Interface Identifiers
– Must begin with a capital letter.
– First alphabet of internal word should also be capitalized.
– Example:
● public class MyClass
● public class Employee
● Variable or Method Identifiers
– Must begin with a small case letter.
– First alphabet of internal word should also be capitalized.
– Example:
● int myNumber
● Constant Identifiers
– Must be specified in upper case.
– _ is used to separate internal words.
– Example:
● final double RATE = 2.6
● Package Identifiers
– Must be specified in small case letters.
– Example:
● Package mypackage.subpackage.x;
Keywords
● Are predefined identifiers meant for specific purpose.
● Reserve words
● All keywords are in lower case.
Ex: case,catch,if,float,int,package,while,do,long
Literals
● Is a value that can be passed to a variable or constant in a program.
● Numeric : binary,octal(0-7,017),hexa
● Boolean: 0’s and 1’s
● Character : enclosed with quotes
● String : zero or more characters
● Null : assigned to object reference variable
Operators
● Using one or more operands
● Unary operator(+)
● Binary operator(/)
● Ternary operator(?:)
Assignment/Arithmetic operator
● Sets the value of a variable to some new value.
● Right to left associativity
● a=b=0
● Ex: +=,-=,|=
● a+=b
Relational operator
● Java return either true or false
● Equal to ==
● Not equal to !=
● Less than <
● Greater than >
● Less than or equal to <=
● Greater than or equal to >=
Boolean logical operators
● Conditional OR ||
● Conditional AND &&
● Logical OR |(TRUE: one is true)
● Logical AND &(FALSE: one is false)
● Logical XOR ^(FALSE: T & T)
● Unary logical NOT ! Ex: bolean a=true; bolean b=false;
To Next Artical We Discuss About Joption Pan Class With Details And example...
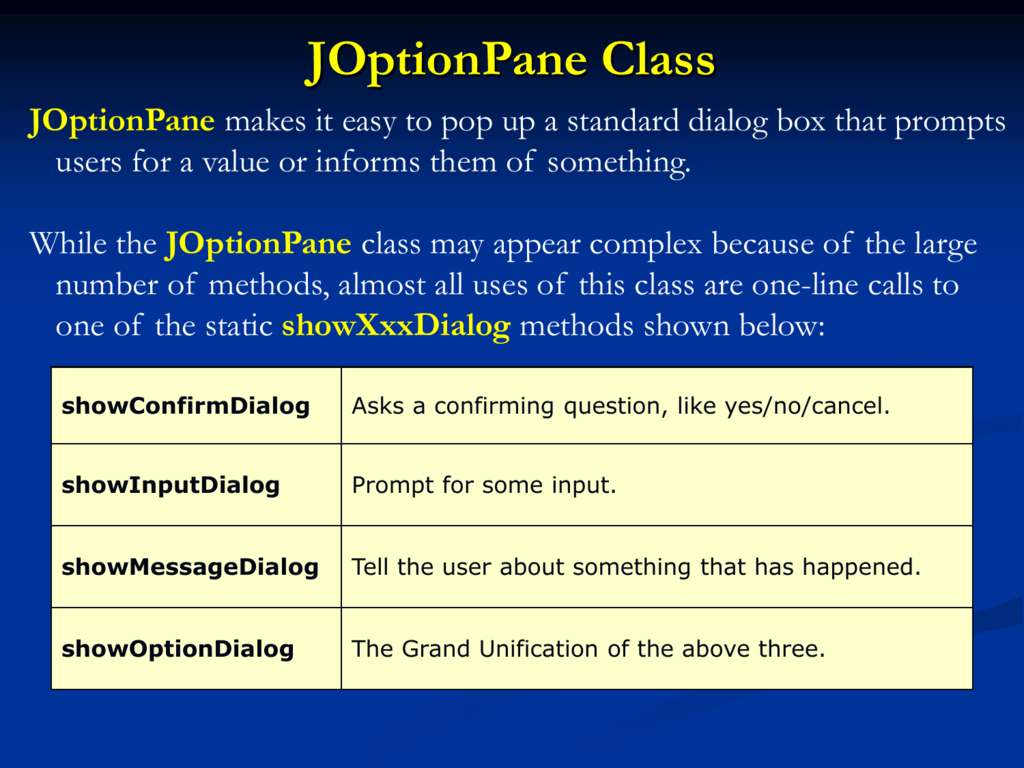
13 Comments
tül perde modelleri
ReplyDeletesms onay
mobil ödeme bozdurma
nft nasıl alınır
ankara evden eve nakliyat
trafik sigortası
dedektor
web sitesi kurma
aşk kitapları
smm panel
ReplyDeleteSmm panel
iş ilanları
İnstagram Takipçi Satın Al
hirdavatciburada.com
BEYAZESYATEKNİKSERVİSİ.COM.TR
SERVİS
Tiktok Jeton Hile
Good content. You write beautiful things.
ReplyDeletevbet
hacklink
hacklink
taksi
mrbahis
korsan taksi
sportsbet
sportsbet
vbet
görükle
ReplyDeletesinop
bodrum +
van
sultanbeyli
LJY
yalova
ReplyDeleteartvin
balıkesir
tuzla
kayseri
4FJ
salt likit
ReplyDeletesalt likit
dr mood likit
big boss likit
dl likit
dark likit
VOBJ16
https://bayanlarsitesi.com/
ReplyDeleteKayseri
Sinop
Kilis
Hakkari
J6BZ
izmir
ReplyDeleteErzurum
Diyarbakır
TekirdaÄŸ
Ankara
XRJ1E
Erzurum
ReplyDeleteistanbul
Ağrı
Malatya
Trabzon
M1İK
whatsapp görüntülü show
ReplyDeleteücretli.show
VMR
9DD9E
ReplyDeleterize rastgele sohbet
agri canli goruntulu sohbet siteleri
ankara canlı sohbet uygulamaları
en iyi rastgele görüntülü sohbet
Afyon En İyi Ücretsiz Sohbet Uygulamaları
kadınlarla sohbet
MuÅŸ Mobil Sohbet Chat
Hatay Kadınlarla Rastgele Sohbet
ücretsiz sohbet uygulaması
F44E5
ReplyDeletedappradar
raydium
layerzero
looksrare
galagames
ledger desktop
ledger live
dexscreener
quickswap
F6495
ReplyDeleteTaşköprü
Orta
Mecitözü
SamandaÄŸ
Eldivan
BaÅŸiskele
Mezitli
Manavgat
Osmancık