Introduction
Java is an Object-Oriented Language. As a language that has the ObjectOriented feature, Java supports the following fundamental concepts −
● Polymorphism
● Inheritance
● Encapsulation
● Abstraction
● Classes
● Objects
● Instance
● Method
● Message Parsing
Flow of Control
● Control flow statements, however, break up the flow of execution by employing decision making, looping, and branching, enabling your program to conditionally execute particular blocks of code.
- Selection (Decision-making) statements (if-then, if-thenelse, switch)
– Looping statements (for, while, do-while)
– Branching statements (break, continue, return)
abc
Selection Statements
● Selection statements allow you to control the flow of program execution on the basis of the outcome of an expression or state of a variable known during runtime.
● Selection statements can be divided into the following categories:
● The if and if-else statements
● The if-else statements
● The if-else-if statements
● The switch statements
Iteration Statements
● Repeating the same code fragment several times until a specified condition is satisfied is called iteration.
● Iteration statements execute the same set of instructions until a termination condition is met.
● Java provides the following loop for iteration statements:
● The while loop
● The for loop
● The do-while loop
● The for each loop
Jump Statements
● Jump statements are used to unconditionally transfer the program control to another part of the program.
● Java provides the following jump statements:
● break statement
● continue statement
● return statement
Classes & Objects
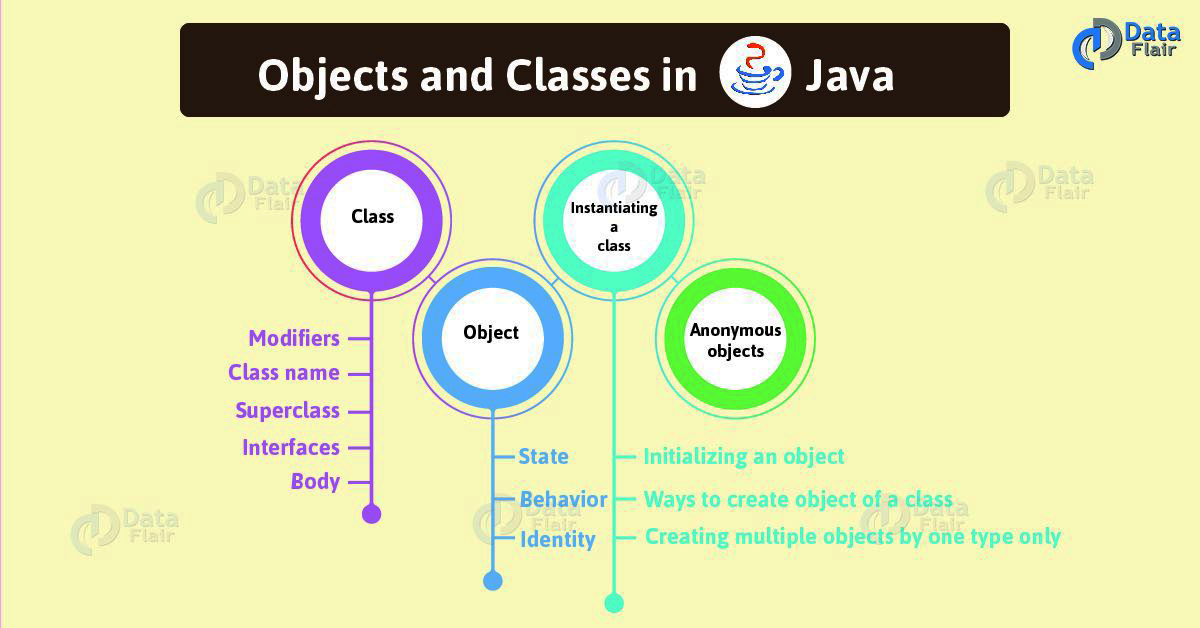
● Object - Objects have states and behaviors.
Example: A dog has states – color, name, breed as well as behaviors – wagging the tail, barking, eating.
● An object is an instance of a class.
● Class - A class can be defined as a template / blueprint that describes the behavior / state that the object of its type support.
● A class is a blueprint or prototype that defines the variables and methods common to all objects of a certain kind,
● We can think of class as a user-defined data types and an object as a variable of that data type that can contain data and methods.
Classes & Objects Example:-
public class Dog
{
String breed;
int age;
String color;
void barking()
{ }
void hungry()
{ }
void sleeping()
{ }
}
Variables:-
● A class can contain any of the following variable types.
● Local variables: Variables defined inside methods, constructors or blocks are called local variables. The variable will be declared and initialized within the method and the variable will be destroyed when the method has completed.
● Instance variables: Instance variables are variables within a class but outside any method. These variables are initialized when the class is instantiated. Instance variables can be accessed from inside any method, constructor or blocks of that particular class.
● Class variables: Class variables are variables declared within a class, outside any method, with the static keyword.
● A class can have any number of methods to access the value of various kinds of methods.
● In the above example, barking(), hungry() and sleeping() are methods.
● A class provides the blueprints for objects. So basically, an object is created from a class. In Java, the new keyword is used to create new objects.
● There are three steps when creating an object from a class:
● Declaration: A variable declaration with a variable name with an object type.
● Instantiation: The 'new' keyword is used to create the object.
● Initialization: The 'new' keyword is followed by a call to a constructor. This call initializes the new object.
NeXt Artical Related Methods AND access modifier in Java
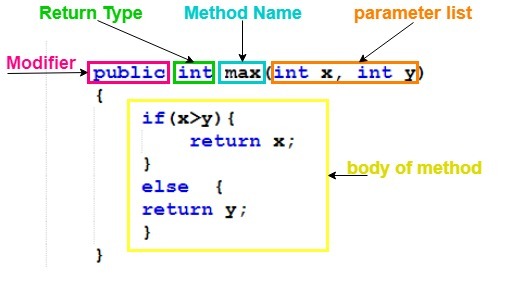
Don't
Forget to Share your Opinion About This post in Comment Section, Your
One Comment Will Not only Make Our day But will Make our Year. And Do
mention Of you have any ideas for our Blog:)
11 Comments
This is really helpful ,thanks to the developers
ReplyDeletekarabük evden eve nakliyat
ReplyDeletebartın evden eve nakliyat
maraÅŸ evden eve nakliyat
mersin evden eve nakliyat
aksaray evden eve nakliyat
8KMDGS
ığdır evden eve nakliyat
ReplyDeletebitlis evden eve nakliyat
batman evden eve nakliyat
rize evden eve nakliyat
niÄŸde evden eve nakliyat
KO3
D06A9
ReplyDeleteAksaray Lojistik
Bolu Parça Eşya Taşıma
Ankara Asansör Tamiri
buy turinabol
Etimesgut Fayans Ustası
Şırnak Parça Eşya Taşıma
Bayburt Parça Eşya Taşıma
Silivri Fayans Ustası
Siirt Evden Eve Nakliyat
2FA14
ReplyDeleteYozgat Lojistik
Edirne Parça Eşya Taşıma
Şırnak Evden Eve Nakliyat
UÅŸak Lojistik
Niğde Şehir İçi Nakliyat
Muş Şehir İçi Nakliyat
Bitlis Parça Eşya Taşıma
Bitlis Şehirler Arası Nakliyat
Ardahan Parça Eşya Taşıma
3A4C7
ReplyDeleteTokat Lojistik
Lbank Güvenilir mi
TekirdaÄŸ Lojistik
Çankırı Lojistik
Elazığ Evden Eve Nakliyat
MaraÅŸ Evden Eve Nakliyat
Edirne Lojistik
Antalya Şehir İçi Nakliyat
Bilecik Şehir İçi Nakliyat
0B1E6
ReplyDeleteCoin Nasıl Kazılır
Btcturk Borsası Güvenilir mi
resimli magnet
Coin MadenciliÄŸi Siteleri
Bitcoin Kazanma Siteleri
resimli magnet
Kripto Para Oynama
Yeni Çıkan Coin Nasıl Alınır
Coin Üretme Siteleri
7C081
ReplyDeleterize telefonda kızlarla sohbet
canlı sohbet odaları
konya canlı ücretsiz sohbet
kırşehir sohbet uygulamaları
urfa sesli sohbet odası
muş random görüntülü sohbet
çanakkale yabancı görüntülü sohbet siteleri
kastamonu kadınlarla ücretsiz sohbet
mersin goruntulu sohbet
A2D14
ReplyDeletemobil proxy 4g
nar sabunu
bitcoin ne zaman yükselir
rastgele canlı sohbet
referans kodu binance
en düşük komisyonlu kripto borsası
tarçın sabunu
okex
binance referans kimliÄŸi nedir
DC8E1
ReplyDeletebitmex
canlı sohbet ücretsiz
kucoin
bibox
kripto para haram mı
telegram kripto para kanalları
rastgele canlı sohbet
referans kimligi nedir
probit
شركة عزل Ø§Ø³Ø·Ø Ø¨Ø¬Ø¯Ø© RdBUwnDKDQ
ReplyDelete