Inheritance in Java

Basic Introduction About Inheritance in Java
- Inheritance in java is a mechanism in which one object acquires all the properties and behaviors of parent object.
- The idea behind inheritance in java is that you can create new classes that are built upon existing classes.
- When you inherit from an existing class, you can reuse methods and fields of parent class, and you can add new methods and fields also.
- Inheritance represents the IS-A relationship, also known as Parent-child relationship.
Understand With Basic Example:-
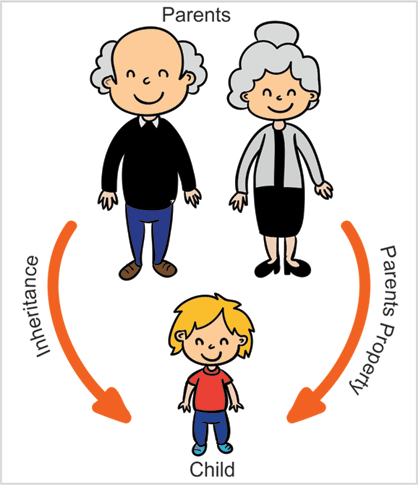
● Why use inheritance in java
– For Method Overriding
– For Code Reusability.
● Syntax of Java Inheritance
class Subclass-name extends Superclass-name
{
//methods and fields
}
● The extends keyword indicates that you are making a new class that derives from an existing class. ● The meaning of "extends" is to increase the functionality.
● In the terminology of Java, a class which is inherited is called parent or super class and the new class is called child or subclass.
Types of Inheritance
● On the basis of class, there can be three types of inheritance in java:- single, multilevel and hierarchical.
● In java programming, multiple and hybrid inheritance is supported through interface only.
● Multiple inheritance is not supported in java through class.

Why Multiple Inheritance is not supported in java???
● To reduce the complexity and simplify the language, multiple inheritance is not supported in java.
● Consider a scenario where A, B and C are three classes. The C class inherits A and B classes.
● If A and B classes have same method and you call it from child class object, there will be ambiguity to call method of A or B class.
● Since compile time errors are better than runtime errors, java renders compile time error if you inherit 2 classes.
● So whether you have same method or different, there will be compile time error now.
What Is Method Overriding

● If subclass (child class) has the same method as declared in the parent class, it is known as method overriding in java.
● In other words, If subclass provides the specific implementation of the method that has been provided by one of its parent class, it is known as method overriding.
Usage of Java Method Overriding
● Method overriding is used to provide specific implementation of a method that is already provided by its super class.
● Method overriding is used for runtime polymorphism.
Rules for Java Method Overriding
● method must have same name as in the parent class
● method must have same parameter as in the parent class.
● must be IS-A relationship (inheritance).
Example of Method Overriding
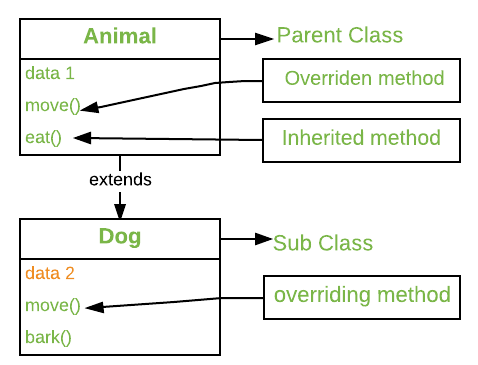
Don't Forget to Share your Opinion About This post in Comment Section, Your One Comment Will Not only Make Our day But will Make our Year. And Do mention Of you have any ideas for our Blog:)
NeXt Artical Related SUper,Final,abstract Keyword in JAva

2 Comments
Nice explain
ReplyDeleteThanks for your comments 👍
Delete