PHP Cookies
- A cookie is often used to identify a user.
- A cookie is a small fle that the server embeds on the user's computer.
- Each time the same computer requests a page with a browser, it will send the
cookie too.
- With PHP, we can both create and retrieve cookie values.
- PHP transparently supports HTTP cookies.
- A cookie is a small fle that the server embeds on the user's computer.
- Each time the same computer requests a page with a browser, it will send the
cookie too.
- With PHP, we can both create and retrieve cookie values.
- PHP transparently supports HTTP cookies.
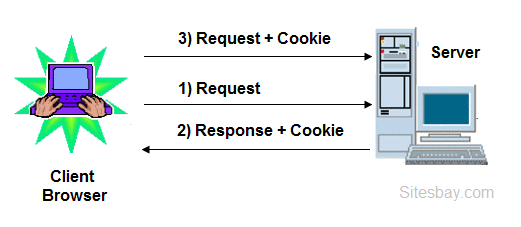
There are three steps involved in identifying returning users.
- Server script sends a set of cookies to the browser.
- For example name, age, or identifcation number etc.
- Browser stores this information on local machine for
future use.
- When next time browser sends any request to web server then it sends those cookies information to the server and server uses that information to identify the
user.
- Server script sends a set of cookies to the browser.
- For example name, age, or identifcation number etc.
- Browser stores this information on local machine for
future use.
- When next time browser sends any request to web server then it sends those cookies information to the server and server uses that information to identify the
user.

Cookie Anatomy:-
Cookies are usually set in an HTTP header.
- A PHP script that sets a cookie might send headers that look
something like this −
- A PHP script that sets a cookie might send headers that look
something like this −
HTTP/1.1 200 OK
Date: Fri, 04 Feb 2000 21:03:38 GMT
Server: Apache/1.3.9 (UNIX) PHP/4.0b3
Set-Cookie: name=xyz; expires=Friday, 04-Feb-07 22:03:38 GMT;
path=/; domain=abc.com
Connection: close
Content-Type: text/html
Date: Fri, 04 Feb 2000 21:03:38 GMT
Server: Apache/1.3.9 (UNIX) PHP/4.0b3
Set-Cookie: name=xyz; expires=Friday, 04-Feb-07 22:03:38 GMT;
path=/; domain=abc.com
Connection: close
Content-Type: text/html
- As you can see, the Set-Cookie header contains a name value pair, a GMT date, a path and a domain. The name and value will be URL encoded.
- The expires feld is an instruction to the browser to "forget" the cookie after the given time and date.
- If the browser is confgured to store cookies, it will then keep this information until the expiry date.
- The expires feld is an instruction to the browser to "forget" the cookie after the given time and date.
- If the browser is confgured to store cookies, it will then keep this information until the expiry date.
Create Cookies With PHP:-
A cookie is created with the setcookie() function.
Syntax
setcookie(name, value, expire, path, domain,secure, httponly);
Only the name parameter is required. All other parameters are optional.
- The setcookie() function must appear BEFORE the <html> tag.
Here is the detail of all the arguments −
- Name:- This sets the name of the cookie and is stored in an environment variable called HTTP_COOKIE_VARS. This variable is used while accessing cookies.
- Value:- This sets the value of the named variable and is the content that you actually want to store.
- Expiry:- This specify a future time in seconds since 00:00:00 GMT on 1st Jan 1970. After this time cookie will become inaccessible. If this parameter is not set
then cookie will automatically expire when the Web Browser is closed.
- Path:- This specifes the directories for which the cookie is valid. A single forward slash character permits the cookie to be valid for all directories.
- Domain:- This can be used to specify the domain name in very large domains and must contain at least two periods to be valid. All cookies are only valid for
the host and domain which created them.
- Security:- This can be set to 1 to specify that the cookie should only be sent by secure transmission using HTTPS otherwise set to 0 which mean cookie
can be sent by regular HTTP.
- Domain:- This can be used to specify the domain name in very large domains and must contain at least two periods to be valid. All cookies are only valid for
the host and domain which created them.
- Security:- This can be set to 1 to specify that the cookie should only be sent by secure transmission using HTTPS otherwise set to 0 which mean cookie
can be sent by regular HTTP.
Simple Demo:-
<?php
$cookie_name = "user";
$cookie_value = "abcd";
setcookie($cookie_name, $cookie_value, time() + 86400, "/");?>
<html>
<body>
<?php
if(!isset($_COOKIE[$cookie_name])) {
echo "Cookie named '" . $cookie_name . "' is not set!";
}
else {
echo "Cookie '" . $cookie_name . "' is set!<br>";
echo "Value is: " . $_COOKIE[$cookie_name];
}?>
</body>
</html>
$cookie_name = "user";
$cookie_value = "abcd";
setcookie($cookie_name, $cookie_value, time() + 86400, "/");?>
<html>
<body>
<?php
if(!isset($_COOKIE[$cookie_name])) {
echo "Cookie named '" . $cookie_name . "' is not set!";
}
else {
echo "Cookie '" . $cookie_name . "' is set!<br>";
echo "Value is: " . $_COOKIE[$cookie_name];
}?>
</body>
</html>
An example that uses setcookie() function to create a cookie named
username and assign the value value John to it. It also specify that the cookie
will expire after 30 days (30 days * 24hours * 60 min * 60 sec).
- setcookie("username", "John", time() +30*24*60*60);
username and assign the value value John to it. It also specify that the cookie
will expire after 30 days (30 days * 24hours * 60 min * 60 sec).
- setcookie("username", "John", time() +30*24*60*60);
Delete a Cookie:-To delete a cookie, use the setcookie() function with an expiration date in the past:
setcookie("user", "", time() - 3600);
Check if Cookies are Enabled:-
- The following example creates a small script that checks whether cookies are
enabled.
First, try to create a test cookie with the setcookie() function, then count the
$_COOKIE array variable:
- The following example creates a small script that checks whether cookies are
enabled.
First, try to create a test cookie with the setcookie() function, then count the
$_COOKIE array variable:
Accessing Cookies with PHP:-
PHP provides many ways to access cookies. Simplest way is to use either $_COOKIE variables.
Following example will access cookies set in above example.
PHP provides many ways to access cookies. Simplest way is to use either $_COOKIE variables.
Following example will access cookies set in above example.
<html>
<body>
<?php
echo $_COOKIE["name"]. "<br />";
echo $_COOKIE["age"] . "<br />";
?>
</body>
</html>
<body>
<?php
echo $_COOKIE["name"]. "<br />";
echo $_COOKIE["age"] . "<br />";
?>
</body>
</html>
isset() function:-
You can use isset() function to check if a cookie is set or not.
<html>
<head>
<title>Accessing Cookies with PHP</title>
</head>
<body>
<?php
if( isset($_COOKIE["name"]))
echo "Welcome " . $_COOKIE["name"] . "<br />";
else
echo "Sorry... Not recognized" . "<br />";
?>
</body>
</html>
You can use isset() function to check if a cookie is set or not.
<html>
<head>
<title>Accessing Cookies with PHP</title>
</head>
<body>
<?php
if( isset($_COOKIE["name"]))
echo "Welcome " . $_COOKIE["name"] . "<br />";
else
echo "Sorry... Not recognized" . "<br />";
?>
</body>
</html>
Retrieving the Cookie value:-
<?php
print_r($_COOKIE);
variable
//output the contents of the cookie array
?>
- Note: $_COOKIE is a PHP built in super global variable.
It contains the names and values of all the set cookies.
The number of values that the
The number of values that the
- $_COOKIE array can contain depends on the memory size set in php.ini.
- The default value is 1GB.
0 Comments